Solochain Integration
For solochains that want to integrate with hyperbridge, a GRANDPA consensus client is provided as a way to track the finality of the Hyperbridge parachain through Polkadot. A consensus relayer is also provided, which is responsible for exchanging finality proofs of cross-chain messages.
Runtime Integration
In your runtime, you should configure Hyperbridge as the coprocessor and add a GRANDPA consensus client to the list of consensus clients. The host state machine should be assigned a unique value for each solochain connected to Hyperbridge. You should also configure a larger block length limit to accommodate for large GRANDPA proofs. The new recommended limit is 8MB
, with a maximum extrinsic limit of 85%.
Every other configuration detail remains unchanged as described in the previous sections
pub RuntimeBlockLength: BlockLength =
BlockLength::max_with_normal_ratio(8 * 1024 * 1024, Perbill::from_percent(85));
parameter_types! {
// The hyperbridge parachain on Polkadot
pub const Coprocessor: Option<StateMachine> = Some(StateMachine::Polkadot(3367));
// The host state machine of this pallet, this must be unique to all every solochain
pub const HostStateMachine: StateMachine = StateMachine::Substrate(*b"solo"); // your unique chain id here
}
impl pallet_ismp::Config for Runtime {
// ...
type Coprocessor = Coprocessor;
// Supported consensus clients
type ConsensusClients = (
// Add the grandpa consensus client here
ismp_grandpa::GrandpaConsensusClient<Runtime>,
);
// ...
}
construct_runtime! {
// ...
Ismp: pallet_ismp
IsmpGrandpa: ismp_grandpa
// ...
}
Whitelisting State Machines
The ismp-grandpa
pallet requires state machines to be whitelisted before any consensus proofs can be accepted, Hyperbridge included. This ensures that solochains are not spammed with consensus proofs of unwanted chains.
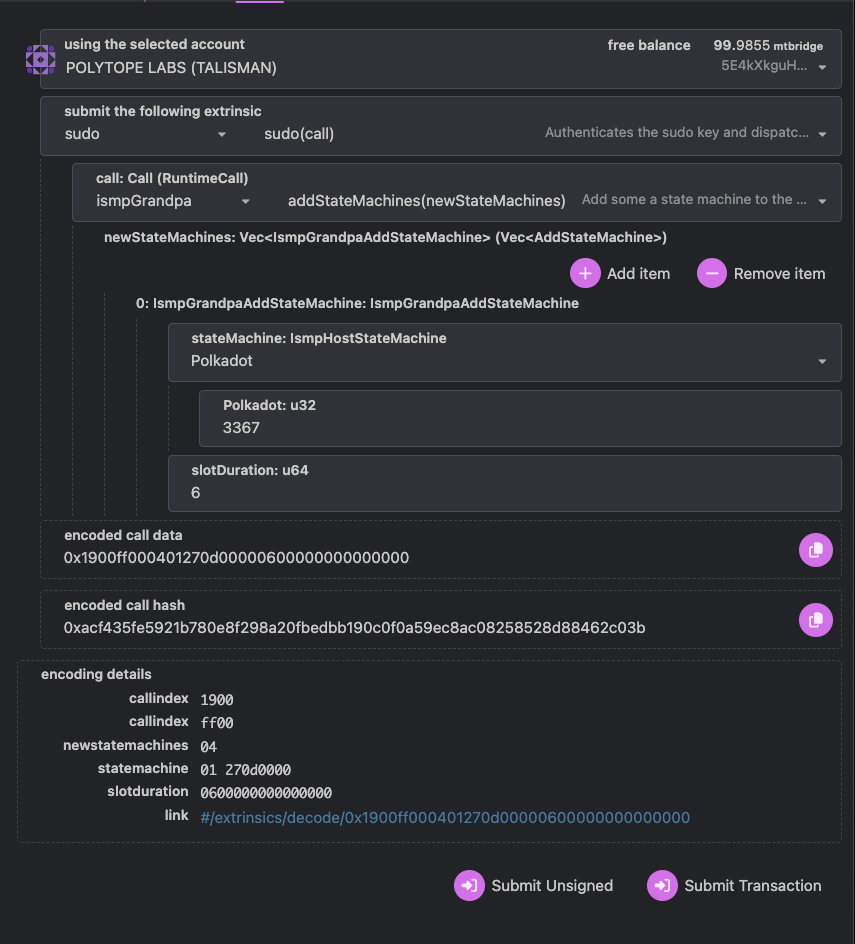
Calls
-
add_state_machines
This adds new state machines which produce GRANDPA consensus proofs to the pallet whitelist, enabling trustless cross-chain communication with the state machines in question.
The arguments are:
1. stateMachine: The state machine identifier for the blockchain to be connected.
2. slotDuration: The slot duration in milliseconds for the blockchain.
-
remove_state_machines
This removes the provided state machines from the pallet whitelist, terminating the trustless connection with the state machines in question. They can always be re-added.
Consensus Relayer
Solochains require an offchain consensus relayer to exchange consensus proofs with Hyperbridge. The Tesseract consensus relayer is built to handle this. This version of the relayer, is different from the publicly available messaging relayer.
Docker
The tesseract consensus relayer is closed-source at this time, but is available as a docker image at the official docker repository polytopelabs/tesseract-consensus
docker pull polytopelabs/tesseract-consensus:latest
Configuring the relayer
The tesseract consensus relayer must be configured to relay consensus messages between a solochain and Hyperbridge. The configuration file is a toml
that should look like:
# Required
[hyperbridge]
type = "grandpa"
[hyperbridge.grandpa]
# Hyperbridge's relay chain websocket RPC
rpc = ""
# Hyperbridge's slot duration
# on Polkadot
# slot_duration = 12000
# on Paseo
slot_duration = 6000
# How frequently to exchange consensus proofs
consensus_update_frequency = 60
# Hyperbridge's paraId on the provided relay chain
# For Paseo Testnet: para_ids = [4009]
# For Polkadot Mainnet: para_ids = [3367]
para_ids = []
[hyperbridge.substrate]
# Hyperbridge's relay chain websocket RPC
rpc_ws = ""
# Hyperbridge's hashing algorithm
hashing = "Keccak"
# Hyperbridge's consensus state id
# For Paseo Testnet: PAS0
# For Polkadot Mainnet: DOT0
consensus_state_id = ""
# Hyperbridge's state machine ID
# For Paseo Testnet: KUSAMA-4009
# For Polkadot Mainnet: POLKADOT-3367
state_machine = ""
# can use any key here
[YourSolochain]
type = "grandpa"
[YourSolochain.substrate]
# Solochains's websocket RPC
rpc_ws = ""
# Hashing can be "Keccak" or "Blake2"
hashing = "Blake2"
# Solochains's consensus state id on Hyperbridge
# should be 4 utf-8 chars chosen by solochain
consensus_state_id = ""
# Solochains's state machine id. eg
state_machine = "SUBSTRATE-myid"
[YourSolochain.grandpa]
# Solochains's websocket RPC
rpc = ""
# Solochains's slot duration
slot_duration = 6000
# How frequently to exchange consensus proofs
consensus_update_frequency = 60
# Any para ids to prove if solochain is actually a relay chain
para_ids = []
[relayer]
maximum_update_intervals = [
# restart if the polkadot consensus client on your solochain is not updated within 3 minutes
[{state_id = "POLKADOT-3367", consensus_state_id = "DOT0"}, 180],
# restart if your solochain consensus client on hyperbridge is not updated within 3 minutes
[{state_id = "SUBSTRATE-myid", consensus_state_id = "MYID"}, 180],
]
Consensus State Initialization
Before running the relayer, you must first initialize Hyperbridge's consensus state on your solochain. The consensus relayer can query Hyperbridge to retrieve this initial state for you. For instance, here's how you can log the consensus state of the Hyperbridge testnet on Paseo:
docker run \
--network=host \
-v /path/to/consensus.toml:/root/consensus.toml \
polytopelabs/tesseract-consensus:latest \
--config=/root/consensus.toml \
log-consensus-state KUSAMA-4009
This should print out a potentially long hex string. Next you'll use this hex string to initialize the Hyperbridge consensus state on your solochain through an extrinsic as shown below.
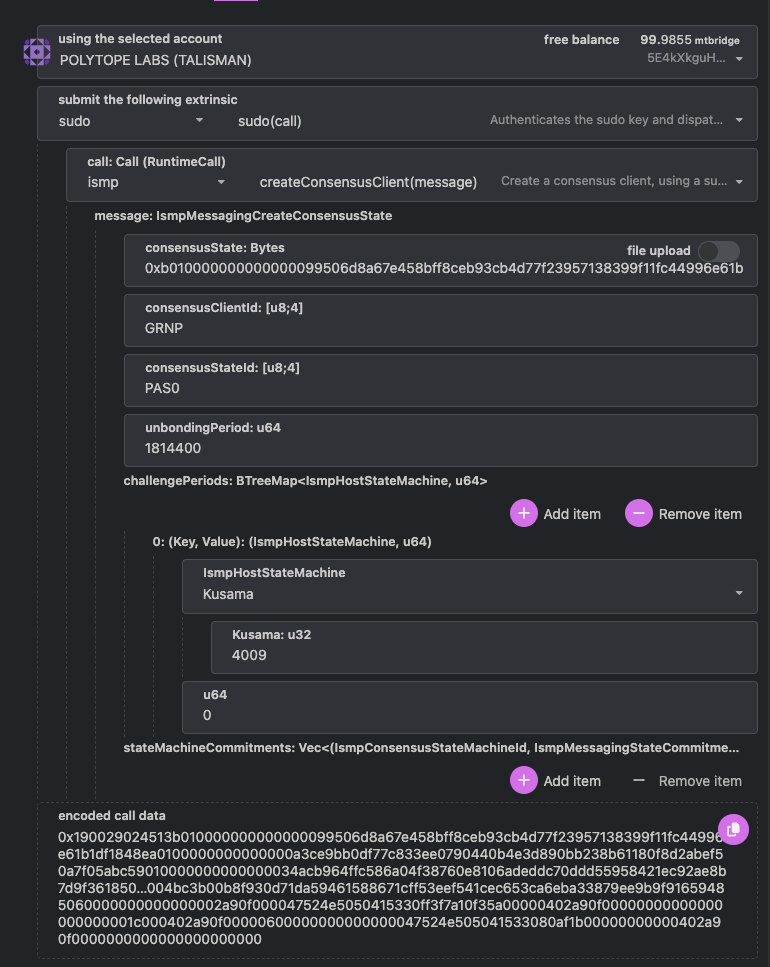
-
consensusState
: This is the initial, trusted consensus state for the Hyperbridge consensus on your solochain. It contains the current and next validator set keys, as well as the latest finalized block. It is the value printed by thelog-consensus-state
subcommand on the tesseract consensus relayer. -
consensusClientId
: This is the consensus client identifier for the GRANDPA consensus client. It will always beGRNP
-
consensusStateId
: This is the consensus state id for Hyperbridge on your solochain, refer to the configuration section on what this value should be. -
unbondingPeriod
: This is the unbonding period for the Hyperbridge relay chain and is used to mitigate long fork attacks. -
challengePeriod
: This is period you want state commitments to stay pending for, even after they have been verified by the consensus client, allowing fishermen to check for byzantine behaviour and submit fraud proofs, if any. Polkadot has high economic security which disincentivizes this sort of behaviour so for Hyperbridge, you can leave set this to zero.
Once this is completed on your solochain, the same will need to also be executed on Hyperbridge. In similar fashion you can log the initial consensus of your solochain and create an issue on our github to be added to the Hyperbridge testnet. On Hyperbridge mainnet, you'll instead submit a governance proposal for this.
docker run \
--network=host \
-v /path/to/consensus.toml:/root/consensus.toml \
polytopelabs/tesseract-consensus:latest \
--config=/root/consensus.toml \
log-consensus-state SUBSTRATE-myid
Running the relayer
Once all consensus states are set up, running the relayer is as easy as:
docker run -d \
--name=consensus \
--restart=always \
--network=host \
-v /path/to/consensus.toml:/root/consensus.toml \
polytopelabs/tesseract-consensus:latest \
--config=/root/consensus.toml
Access it's logs via
docker logs -f consensus -n=${maximum_number_of_previous_log_lines}
You will of course need to pair this with the messaging relayer which actually relays cross-chain messages.
Miscallenous
Slot Numbers
The GRANDPA consensus client relies on digest items in the header to communicate the current slot number. These digest items must be identified as either BABE
or AURA
. Solochains using custom block production algorithms must include the AURA
digest item to ensure compatibility with the GRANDPA consensus client for producing valid consensus proofs.